Efficiently processing data from files is crucial in many Java applications. Understanding how to read files and create a 2D array is a fundamental skill for handling tabular data, image processing, or any scenario involving two-dimensional datasets. This process involves several key steps, from selecting the appropriate file input method to correctly parsing the data and constructing the array. The choice of data structures and error handling also significantly impacts the robustness and performance of the solution. Mastering this technique allows developers to build more sophisticated and efficient programs capable of manipulating complex datasets.
The ability to read data from files and structure it into a two-dimensional array is a cornerstone of many data processing tasks in Java. This technique enables efficient manipulation and analysis of structured information. Imagine processing a CSV file containing sales data for different products across various regions. Representing this data as a 2D array allows for easy access to individual sales figures based on product and region. This structured approach simplifies calculations, aggregations, and other analytical operations that would be significantly more complex with unstructured data. The efficiency of this approach scales well with increasing dataset sizes.
Beyond simple data representation, constructing a 2D array from file data allows for the application of various matrix operations. This is particularly valuable in fields like image processing, where pixel data can be naturally represented as a 2D array, facilitating operations like filtering, transformation, and color manipulation. Moreover, the use of 2D arrays simplifies the implementation of algorithms that require accessing elements based on their row and column indices, ensuring a clear and intuitive data structure. Efficient memory management within the 2D array contributes to the overall performance of the application.
Furthermore, the method used to read and parse the file data significantly impacts the overall efficiency and robustness of the program. For instance, handling potential exceptions, such as the file not being found or containing unexpected data formats, is crucial for preventing program crashes. Employing appropriate error-handling mechanisms ensures the reliability of the data processing pipeline and prevents unexpected behavior. This also contributes to a cleaner and more maintainable codebase. The judicious choice of file reading techniques and error management is key to creating a reliable and efficient solution.
Reading Files and Creating 2D Arrays in Java
This section details the process of reading data from a file and populating a two-dimensional array in Java. The process typically involves opening a file, reading its content line by line, parsing each line to extract relevant data, and finally, populating the 2D array with the extracted information. Effective error handling is crucial throughout this process to ensure robust operation. Different file formats will require different parsing strategies, and selecting the optimal approach for the target file format is essential for efficient processing. The choice of data type for the array elements also impacts the overall memory usage and efficiency.
-
Open the File:
The first step involves using a `FileReader` or `BufferedReader` to open the file. Ensure appropriate error handling (e.g., using `try-catch` blocks) to manage potential exceptions like `FileNotFoundException`.
-
Read the File Line by Line:
Use a `BufferedReader` and a loop to read the file line by line. Each line typically represents a row in the 2D array.
-
Parse Each Line:
Split each line into individual elements using methods like `String.split()`. Convert these elements to the appropriate data type (e.g., integer, double) using methods like `Integer.parseInt()` or `Double.parseDouble()`.
-
Populate the 2D Array:
Create a 2D array of the appropriate size and data type. Populate the array with the parsed elements, ensuring that the row and column indices are correctly mapped to the data.
-
Close the File:
After processing, close the file using the `close()` method to release resources. This is crucial for proper resource management.
Tips for Efficient File Reading and 2D Array Creation
Optimizing the file reading and 2D array creation process can significantly improve the efficiency and maintainability of your Java code. Careful consideration of data structures, error handling, and resource management leads to more robust and performant applications. Understanding the characteristics of your input data and choosing appropriate parsing strategies significantly impacts overall processing speed.
Employing best practices for exception handling and resource management is vital for building reliable applications. This involves using `try-with-resources` to automatically close files and other resources, minimizing the risk of resource leaks. Consider using buffered input streams to improve performance by reducing the number of disk reads.
-
Use Buffered Input Streams:
Employ `BufferedReader` to improve I/O performance by buffering reads from the file, reducing the number of disk accesses.
-
Handle Exceptions Gracefully:
Implement proper exception handling using `try-catch` blocks to handle potential errors like `FileNotFoundException` or `IOException`.
-
Pre-allocate Array Size:
If possible, determine the array size beforehand to avoid the overhead of resizing during population. This is particularly helpful for larger datasets.
-
Choose Appropriate Data Types:
Select the most appropriate data type for your array elements (e.g., `int`, `double`, `String`) to minimize memory usage and improve efficiency.
-
Employ `try-with-resources` :
Use `try-with-resources` to ensure that resources, such as file streams, are automatically closed, even in case of exceptions.
-
Consider using Scanner:
The `Scanner` class can simplify parsing of data from the file, especially when dealing with different delimiters.
-
Optimize String Processing:
If string manipulation is involved, consider using more efficient techniques like `StringBuilder` instead of repeated string concatenation.
Efficiently managing memory is vital, especially when dealing with large files. Pre-allocating the 2D array to its final size avoids dynamic resizing, which can be computationally expensive. Selecting appropriate data types for the array elements minimizes memory footprint. Careful consideration of these factors contributes to the overall performance and scalability of the application. Efficient memory management is especially crucial when processing large datasets, as it can prevent OutOfMemoryErrors.
Robust error handling is paramount. Properly handling exceptions such as `FileNotFoundException` and `IOException` prevents program crashes and ensures that the application gracefully handles unexpected situations. Employing techniques such as `try-catch` blocks and using `finally` blocks to ensure resource cleanup are essential components of a well-structured and resilient program. Implementing thorough error checking helps maintain the reliability and stability of the overall application.
The selection of appropriate data structures significantly impacts the overall design and efficiency. Using 2D arrays is suitable for many scenarios, but for particularly large datasets, consider alternatives like specialized data structures that might offer better performance characteristics depending on the nature of the data processing tasks to be performed. Selecting the right data structure impacts efficiency and memory management, directly affecting the performance of the application.
Frequently Asked Questions
This section addresses frequently encountered questions regarding reading files and creating 2D arrays in Java. Understanding these common issues and their solutions improves developer proficiency and problem-solving capabilities. Addressing these questions proactively helps prevent common pitfalls and ensures the successful implementation of data processing tasks.
-
How do I handle files with varying numbers of elements per line?
Use a dynamically sized ArrayList of ArrayLists to accommodate lines with differing element counts. This provides flexibility in handling inconsistent data.
-
What is the best way to handle empty lines in a file?
Skip empty lines using appropriate checks within your file reading loop. You can use `line.trim().isEmpty()` to identify and ignore empty lines efficiently.
-
How do I handle different delimiters in the file (e.g., commas, tabs)?
Use the `String.split()` method with the appropriate delimiter as the argument. This allows for flexible handling of varied file formats.
-
What if my file is very large and doesn’t fit in memory?
For exceptionally large files, consider processing the file in chunks or using external sorting techniques to avoid memory issues.
-
How can I improve the performance of my file reading code?
Using buffered input streams and optimizing string processing are crucial performance enhancements. Pre-allocating arrays and minimizing unnecessary operations can also significantly improve efficiency.
-
What are some common errors encountered when working with files and 2D arrays?
Common errors include `FileNotFoundException`, `IOException`, `ArrayIndexOutOfBoundsException`, and `NumberFormatException`. Proper exception handling is crucial to prevent program crashes.
Successfully implementing file reading and 2D array creation involves understanding and applying the principles of efficient resource management, error handling, and data structure selection. Choosing appropriate data types and optimizing string processing are also critical factors impacting performance and scalability. Careful planning and design contribute to creating robust and efficient applications.
The process of reading files and populating 2D arrays in Java demands a methodical approach that encompasses careful file handling, robust data parsing, and efficient memory management. Addressing potential issues proactively through effective error handling enhances the reliability of the solution. Understanding different file formats and selecting the appropriate parsing strategy for each is key to building versatile and efficient applications.
Mastering this technique unlocks a wide range of possibilities for manipulating and analyzing data efficiently. The ability to seamlessly integrate data from various sources into structured 2D arrays facilitates numerous applications in areas ranging from data analysis to image processing and beyond. This skill forms a cornerstone for building robust and performant data-centric applications in Java.
In conclusion, the ability to effectively read files and create 2D arrays is a fundamental skill in Java programming, offering significant advantages in data manipulation and analysis. By following best practices and understanding common challenges, developers can create robust and efficient applications capable of handling a wide variety of data processing tasks.
Youtube Video Reference:
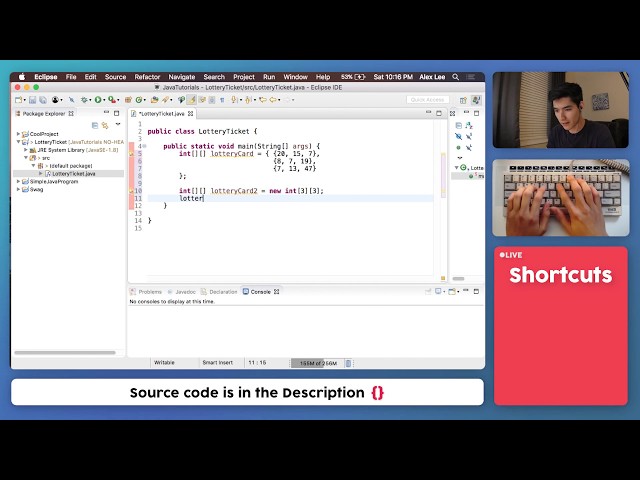