Determining whether a string represents a numerical zero is a common task in Python programming. How to check string equals zero in python efficiently and accurately is crucial for data validation, conditional logic, and preventing errors. This involves understanding the nuances of string comparisons and employing appropriate techniques to avoid common pitfalls. The process can be surprisingly subtle, so a clear understanding of the different approaches and their implications is vital for robust code. This article provides a comprehensive guide to successfully handling this situation.
The straightforward approach involves a direct comparison using the equality operator (`==`). However, this method alone might not suffice. A string representation of ‘0’ is distinct from an integer 0. Simply comparing ‘0’ == 0 will return `False`. Therefore, more sophisticated methods are needed, frequently involving type conversion or more nuanced string manipulation techniques. Proper error handling should be included to manage scenarios where the input string isn’t even a valid numerical representation. Ignoring such edge cases can lead to unexpected program behavior or crashes. The choice of method depends on the context and the desired level of strictness in validation. Some scenarios may tolerate whitespace, while others demand absolute precision.
Another important aspect is the handling of whitespace. A string like ” 0 ” contains a leading and trailing space, which a simple equality check against ‘0’ would fail to detect. Therefore, appropriate techniques, such as the `strip()` method to remove leading and trailing whitespace, are often necessary before performing the comparison. Furthermore, the need to handle negative zero representations (‘-0’) should also be addressed. While numerically equivalent to 0, they are different strings and require specific consideration depending on application requirements. A robust solution should account for these variations to ensure accurate results.
Finally, error handling plays a significant role. Attempting to convert a non-numeric string to an integer will trigger a `ValueError`. Therefore, robust solutions include `try-except` blocks to gracefully handle such potential exceptions, preventing program crashes and ensuring more reliable code. By anticipating and handling potential errors, a program becomes significantly more resilient to unexpected input, and maintains a smooth user experience regardless of data quality. The implementation should prioritize clarity and maintainability so as to allow for easy understanding and modification later.
How to check if a string represents zero in Python?
Checking if a string represents the numerical value zero requires careful consideration of several factors. Simple equality checks might be insufficient due to potential leading/trailing whitespace or the difference between string and numeric types. Therefore, a robust approach requires a combination of string manipulation and error handling to ensure accuracy and reliability. Choosing the right method depends largely on the strictness required in the validation process. This section will outline several efficient methods to determine if a string equates to zero, clarifying the strengths and limitations of each.
-
Method 1: Direct Comparison after Stripping Whitespace
This method first removes leading and trailing whitespace from the string using the `strip()` method, then compares the resulting string to ‘0’. This approach handles strings with extra spaces but still only compares against a string representation of zero.
-
Method 2: Type Conversion and Comparison
This method attempts to convert the string to an integer using `int()`. If successful, it compares the integer to 0. This approach handles string representations of integers, including negative zero. However, it will fail if the string contains non-numeric characters and raises a `ValueError`, requiring a `try-except` block for proper error handling.
-
Method 3: Using `Decimal` for Floating-Point Precision
For situations involving floating-point numbers, use the `decimal.Decimal` module to account for potential precision issues. This converts the string to a decimal object and then compares it to Decimal(‘0’). This is critical for ensuring precise comparison when dealing with floating-point representations of zero which might not be exactly equal to 0 due to how floating-point numbers are stored in memory.
-
Method 4: Regular Expression Matching
A more sophisticated method employs regular expressions. This technique allows for a highly customizable comparison, handling variations like leading/trailing whitespace, signs, and even allowing for scientific notation. However, this method has a steeper learning curve compared to the previous approaches.
Tips for Effectively Checking String Representations of Zero
While the methods above provide solid solutions, several additional tips can help to refine the process and enhance code robustness and clarity. Considering these factors contributes to cleaner, more efficient, and less error-prone code. This section emphasizes the subtle details often overlooked and provides strategies for optimizing the validation process.
Careful consideration should always be given to potential edge cases and the need for comprehensive error handling, as a failure to account for these scenarios can significantly impact application reliability.
-
Always Handle Exceptions:
Wrap type conversion attempts (like `int()`) within `try-except` blocks to gracefully handle cases where the input string is not a valid representation of a number. This prevents runtime errors and ensures a more stable application.
-
Consider Whitespace:
Always account for potential leading or trailing whitespace. Use the `strip()` method to remove these before comparison to avoid false negatives.
-
Handle Negative Zero:
Decide whether to treat ‘-0’ as equivalent to ‘0’. If so, ensure your approach considers this scenario. If not, adjust your comparison logic accordingly.
-
Choose the Right Data Type:
If dealing with floating-point numbers, use `decimal.Decimal` for increased accuracy. Standard floats might not always provide an exact representation of zero due to inherent limitations of floating-point arithmetic.
-
Document Your Approach:
Clearly document the chosen method and its rationale within your code. This is crucial for maintainability and understanding for future developers or yourself.
-
Use Assertions (for Development):
During development, use `assert` statements to verify that the input is a valid numeric string before processing. This helps in early error detection and debugging.
-
Unit Testing:
Thoroughly test your function with various inputs, including valid numeric strings, invalid strings, and strings with whitespace. Use a unit testing framework like `pytest` to systematically check for correctness.
The robustness of a program is significantly enhanced by carefully handling all possible scenarios. While a direct comparison might seem sufficient at first glance, a thorough examination reveals a need for more comprehensive techniques. The importance of this seemingly simple task underscores the need for meticulous attention to detail in software development. A robust solution must consider not only the direct comparison but also whitespace, potential errors, and the implications of different numerical representations. Such an approach leads to reliable code that avoids unexpected failures or incorrect behavior. It’s important to stress that handling edge cases is not simply an optional refinement, but a crucial aspect of good programming practice.
Furthermore, the selection of the appropriate method is dependent upon the particular requirements of the program. Different applications might necessitate different levels of precision or tolerance for variations in string representation. Therefore, a flexible approach that can adapt to changing needs is preferable. Prioritizing clarity in the implementation enhances both maintainability and readability, reducing the likelihood of errors in the long run. By adhering to best practices in exception handling and documentation, a programmer can develop more trustworthy and maintainable code.
In conclusion, the seemingly simple task of determining whether a string represents zero in Python necessitates a more sophisticated approach than a simple equality check. A robust solution accounts for variations in string representation, incorporates appropriate error handling, and considers the context of the application. Adopting a methodical approach to these considerations leads to better code quality and program reliability. The focus on clarity, maintainability, and comprehensive testing is essential for developing high-quality software.
Frequently Asked Questions
This section addresses common questions that arise when dealing with string comparisons related to zero. The goal is to provide clarification and further solidify the concepts discussed in this article. These commonly encountered scenarios often highlight areas where less thorough approaches might fall short.
-
How do I handle strings with leading/trailing spaces?
Use the `strip()` method to remove whitespace before comparing the string. For example: `if my_string.strip() == ‘0’:`
-
What if my string contains non-numeric characters?
Use a `try-except` block to handle potential `ValueError` exceptions during type conversion (e.g., `int()`). This prevents program crashes and allows for graceful error handling.
-
Why should I use `decimal.Decimal` instead of standard floats?
Standard floats might not accurately represent zero due to floating-point precision issues. Using `decimal.Decimal` ensures greater accuracy, especially when comparing floating-point representations of zero.
-
Can I use regular expressions for this task?
Yes, regular expressions can provide a flexible and powerful solution, enabling the handling of various string representations of zero, including scientific notation and different formats. However, this approach increases complexity.
-
How can I make my code more robust?
Implement comprehensive error handling using `try-except` blocks, handle whitespace appropriately, document your approach clearly, and conduct thorough testing using a unit testing framework. These strategies enhance code reliability and maintainability.
The importance of this seemingly simple task cannot be overstated. Effective handling of these string comparisons is fundamental for the reliability and robustness of many Python programs. The potential for errors is substantial if a naive approach is adopted. The focus on comprehensive error handling, precise type conversion, and thoughtful selection of validation methods helps prevent program failures and enhances the overall quality of the code.
By integrating the tips and methods outlined in this document, a robust and reliable system for checking string representations of zero can be implemented. This approach ensures that your programs are less prone to failures and more easily maintainable. This, in turn, translates to more efficient and dependable applications.
In summary, understanding the subtleties of how to appropriately check whether a string represents zero in Python is crucial for developing resilient and reliable code. The various approaches presented offer a range of options, enabling programmers to select the most suitable method based on their specific needs and context. Adhering to best practices in error handling and documentation guarantees a more maintainable and error-free development process.
Therefore, a thorough understanding of how to check string equals zero in python is a fundamental skill for any Python developer seeking to write robust and reliable applications.
Youtube Video Reference:
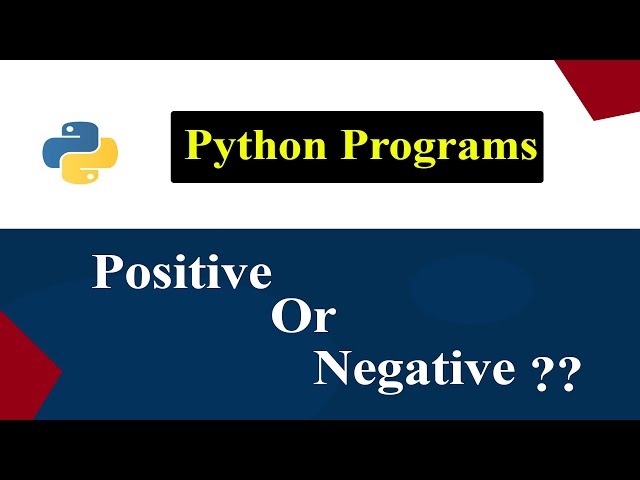