Understanding how to access error case structures is fundamental to effective debugging and software development. The method for accessing these structures varies significantly depending on the programming language, the specific error handling mechanisms used, and the environment in which the application runs. This exploration will cover several common approaches and strategies for examining error details, offering insights into efficient troubleshooting and improving code robustness. Accessing and interpreting this information is crucial for identifying the root cause of problems and implementing appropriate solutions. This article provides a comprehensive guide for navigating the complexities involved.
Error case structures, broadly defined, encapsulate information about exceptions, failures, or unexpected events that occur during program execution. They typically include details such as the error type, a description of the problem, the location where the error occurred (e.g., file, line number), and potentially a stack trace detailing the sequence of function calls leading to the error. This data is invaluable for developers in pinpointing the source of issues and implementing corrective actions. Efficiently accessing and interpreting this information is a critical skill for any programmer.
The level of detail provided within an error case structure can vary greatly. Some systems provide minimalistic error messages, while others offer rich diagnostic information, including variable values at the point of failure, which can dramatically expedite debugging. Therefore, familiarity with the tools and techniques available within a given development environment is key. Understanding the error structure directly contributes to faster resolution of problems, reduced downtime, and a more reliable application.
Different programming paradigms offer distinct approaches to error handling. Object-oriented languages often utilize exception handling mechanisms, which provide structured ways to catch and process errors. Procedural languages may rely on return codes or error flags to signal problems. Regardless of the paradigm, the core principle remains the same: the ability to access and interpret the information contained within an error case structure is essential for successful debugging. The techniques for extracting this information will vary according to the specific language and system used.
How to Access Error Case Structures?
Accessing error information depends heavily on the context. The operating system, programming language, and even the specific libraries utilized all influence how errors are reported and accessed. This section details several common approaches. Understanding the nuances of each method empowers developers to diagnose and resolve issues effectively and efficiently. The process often involves examining logs, utilizing debugging tools, or directly interrogating exception objects.
-
Examine Log Files:
Many systems record errors in log files. These files often contain timestamps, error codes, and descriptive messages. The location of these logs varies, depending on the system and application. Examining these log files is often the first step in troubleshooting. Analyzing the log entries will generally yield specific clues as to the error source.
-
Use a Debugger:
Debuggers allow developers to step through code execution line by line, examine variable values, and inspect the program’s state at various points. When an error occurs, a debugger typically provides detailed information about the error, including the stack trace, which is crucial for pinpointing the root cause.
-
Inspect Exception Objects (Object-Oriented Languages):
In object-oriented languages, errors are frequently handled using exceptions. Exception objects contain information about the error. By catching these exceptions, developers can access the stored data to gain insights into the cause of the failure. This access often involves inspecting properties of the exception object.
-
Check Return Codes (Procedural Languages):
Procedural languages often rely on return codes to indicate success or failure. A non-zero return code usually signals an error. The documentation of the relevant functions will typically define the meaning of each return code. Understanding what each code means is critical for accurate analysis.
Tips for Effectively Accessing Error Case Structures
Efficiently accessing and interpreting error information is crucial for swift problem resolution. The following guidelines enhance the process, aiding in faster identification of error sources and promoting more robust software.
Developing a systematic approach to error analysis saves valuable time and resources. These tips promote a more structured and effective troubleshooting methodology.
-
Utilize a Consistent Logging Strategy:
Implement a consistent logging system across the application. This includes recording timestamps, error levels (e.g., debug, warning, error), and comprehensive error messages. Well-structured logs are essential for tracing errors and understanding the context in which they occurred.
-
Employ a Version Control System:
Utilize a version control system (like Git) to track changes to code. This makes it easier to revert to earlier versions if a change introduces an error. Tracking revisions provides a trail to analyze when a problem might have been introduced.
-
Leverage Debugging Tools Effectively:
Familiarize yourself with the debugging tools available within your development environment. Learn how to set breakpoints, step through code, inspect variables, and examine the call stack. These tools are invaluable for understanding the execution flow and isolating the source of errors.
-
Use Exception Handling Appropriately:
In object-oriented programming, utilize exception handling mechanisms effectively. Catch specific exceptions and handle them gracefully. Avoid generic exception handlers that mask the underlying issues.
-
Consult Relevant Documentation:
Always refer to the documentation of libraries and frameworks used in your project. The documentation often provides detailed information on error codes and how to interpret error messages.
-
Employ Automated Testing:
Implement automated testing to identify potential errors early in the development cycle. Comprehensive testing helps prevent errors from reaching production and simplifies debugging when problems occur.
-
Regularly Review Logs:
Develop a routine for regularly reviewing application logs. This helps identify recurring errors or emerging problems before they escalate. Proactive monitoring is key to preventing larger scale issues.
Effective error handling is paramount to producing reliable software. Understanding the data contained within error case structures significantly impacts the speed and effectiveness of problem resolution. Proactive monitoring coupled with robust error handling prevents small issues from becoming major problems.
A well-structured error handling strategy not only aids in quicker debugging but also contributes to a more user-friendly experience. When errors are handled gracefully, users receive informative messages and the application remains stable, minimizing disruptions. This positive user experience is vital for application success.
Ultimately, the ability to access and interpret error case structures is a core competency for any developer. By mastering these techniques, developers can dramatically improve code quality, reduce downtime, and create more robust and reliable applications. Proficiency in this area directly translates to better software and more efficient development workflows.
Frequently Asked Questions about Accessing Error Case Structures
This section addresses common questions related to accessing and interpreting error information, providing practical guidance for developers facing common challenges. Understanding these common issues promotes faster problem resolution and contributes to better overall development practices.
-
What if I cannot find any error messages?
If you’re unable to locate error messages, check the following: ensure logging is enabled; verify the log file location; check for permissions issues preventing access; inspect the application’s configuration for error reporting settings. Sometimes, error messages are suppressed or redirected; carefully review the application’s settings to identify potential misconfigurations.
-
How can I decipher cryptic error codes?
Cryptic error codes often require consulting the relevant documentation for the system, library, or API in use. The documentation will generally provide a detailed explanation of each error code. Online searches, using the code as a keyword, can often yield helpful information.
-
What is a stack trace, and how is it useful?
A stack trace is a sequence of function calls that led to an error. It shows the path of execution, indicating the sequence of events that resulted in the failure. This sequence is essential for identifying the root cause of the problem, often pinpointing the specific line of code where the error originated.
-
How do I improve error messages in my own code?
Provide clear, informative error messages that include context. Use descriptive messages rather than generic ones. Consider including the relevant variables or data contributing to the error. Well-crafted error messages significantly aid in debugging.
-
What are some common pitfalls to avoid when handling errors?
Common pitfalls include neglecting to handle exceptions properly, using generic exception handlers, and failing to log errors adequately. Ignoring error handling can lead to application crashes and data loss. Careful and comprehensive error handling is critical.
Effective error handling is an ongoing process, requiring a combination of proactive measures and reactive responses. Regularly reviewing error handling strategies and updating them as needed ensures the continuous improvement of application robustness.
Developing robust error handling mechanisms isn’t just about fixing problems; it’s about building a more resilient system capable of gracefully handling unexpected events. This resilience translates to a better user experience and improved overall application stability.
In conclusion, understanding how to effectively access and interpret error case structures is a crucial skill for software developers. By mastering the techniques and strategies outlined in this article, developers can significantly improve their ability to debug issues, enhance code quality, and create more reliable applications.
Youtube Video Reference:
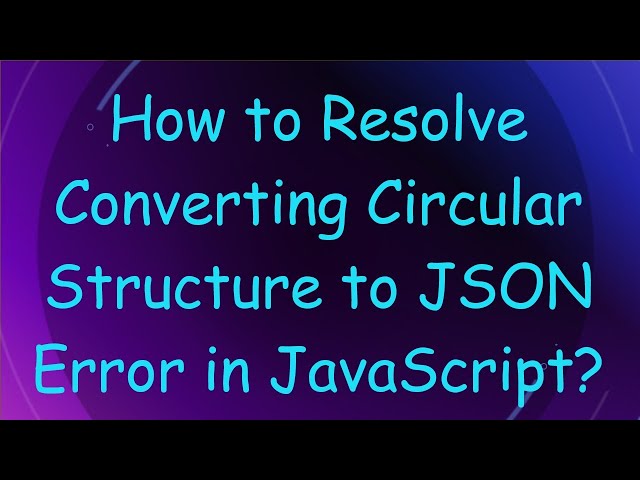