Understanding the process of how to create your own JS library offers significant advantages for developers. Creating a custom library allows for the encapsulation of reusable code, promoting consistency and reducing redundancy across projects. This ultimately enhances efficiency and improves code maintainability. Well-structured libraries also enhance collaboration within development teams. Furthermore, creating a personal library can lead to a deeper understanding of JavaScript and software design principles. Finally, a well-designed library can be a valuable asset in a developer’s portfolio, showcasing their skills and expertise.
The development of a JavaScript library involves careful planning and execution. A clear definition of the library’s purpose and functionality is paramount before beginning the coding process. This includes identifying the specific tasks or problems the library aims to solve. Modular design is also crucial, allowing for individual components to be easily tested and maintained independently. Thorough testing throughout development is essential to ensure robustness and reliability. This process helps identify and correct errors before release. The selection of appropriate documentation methods is vital for easy usability. Well-documented code enhances understanding and simplifies future modifications or extensions.
Version control is a critical aspect of library development, providing a history of changes and enabling easy rollback to previous versions if necessary. This is typically accomplished using Git or similar version control systems. Proper error handling is also crucial for creating a robust and reliable library, ensuring graceful degradation when unexpected situations arise. Consideration of browser compatibility is a crucial factor that should be addressed in the design phase to ensure wide usage and minimize potential issues. Performance optimization techniques are beneficial in ensuring efficiency and speed. This might involve techniques like code minification or using optimized data structures.
Deployment strategies need careful consideration to ensure the library is easily accessible to users. This often involves hosting the library on platforms like npm, GitHub, or a similar repository. Regular updates and maintenance are crucial for addressing any bugs, incorporating new features, and maintaining compatibility with evolving JavaScript standards. This continuous improvement loop enhances the librarys value and longevity. Community engagement, while optional, can greatly benefit library development through feedback and contributions. Active participation in relevant online communities and forums can increase the reach and impact of a custom library.
How to Create Your Own JS Library?
Developing a JavaScript library is a structured process requiring careful planning and execution. It begins with a well-defined purpose and scope, identifying the specific problems or tasks the library will address. This involves thorough analysis of potential use cases and anticipated requirements. The next step involves designing the librarys architecture, breaking it down into manageable, modular components for easier maintenance and testing. A robust testing strategy is crucial throughout development to identify and resolve any issues early on. Efficient documentation should be created to facilitate easy understanding and usage of the library. Finally, deploying and maintaining the library requires careful consideration of accessibility and continuous improvement.
-
Define Scope and Purpose:
Clearly articulate the library’s goals, functionalities, and target audience. This initial planning phase is crucial for guiding the entire development process.
-
Modular Design:
Break down the library into smaller, independent modules. This improves code organization, maintainability, and reusability.
-
Choose a Module System:
Select a suitable module system (e.g., CommonJS, AMD, ES modules) to manage dependencies and organize code effectively.
-
Write the Code:
Implement the core functionality, adhering to best practices and coding conventions for maintainability and readability.
-
Thorough Testing:
Write comprehensive unit and integration tests to ensure functionality and prevent errors. Use testing frameworks like Jest or Mocha.
-
Documentation:
Create clear and concise documentation explaining how to use the library, including examples and API references.
-
Version Control:
Utilize a version control system (like Git) to track changes, manage different versions, and collaborate effectively.
-
Deployment:
Publish your library using a package manager like npm or yarn, making it easily accessible to others.
Tips for Creating a Successful JS Library
Creating a highly effective JavaScript library involves more than just writing functional code; it requires strategic planning and consideration of various factors that contribute to its usability, maintainability, and longevity. Understanding these key elements will greatly increase the chances of creating a library that is both useful and well-received within the developer community. Key aspects include modular design, thorough documentation, and comprehensive testing. The choice of a suitable module system and version control strategy is equally important for efficient collaboration and management of different versions of the library.
Focusing on performance optimization, error handling, and browser compatibility ensures robustness and a positive user experience. Furthermore, ongoing maintenance, including bug fixes, security updates, and new features, extends the life and value of the library, building a reputation of quality and reliability within the community of users.
-
Modular Design:
Divide the library into smaller, independent modules for easier maintenance and testing. This also enhances reusability.
-
Comprehensive Documentation:
Provide detailed documentation with clear examples and API references to make the library easy to use.
-
Robust Testing:
Implement thorough unit and integration tests to ensure code quality and catch errors early on.
-
Version Control:
Use Git or a similar system for managing code changes, tracking versions, and collaborating effectively.
-
Performance Optimization:
Optimize the library’s performance to minimize loading times and ensure efficient execution.
-
Error Handling:
Include robust error handling to gracefully manage unexpected situations and provide helpful error messages.
-
Browser Compatibility:
Ensure compatibility across major browsers to reach a wider audience. Conduct thorough cross-browser testing.
-
Regular Maintenance:
Plan for ongoing maintenance, including bug fixes, security updates, and new features, to ensure longevity.
Effective library design prioritizes simplicity and readability. Avoid unnecessary complexity and strive for code that is easily understood and maintained. A well-designed API simplifies interactions and minimizes the learning curve for users. Consider the potential use cases and anticipate how others might integrate the library into their projects. This user-centric approach improves adoption and satisfaction. A consistent coding style across the librarys components enhances readability and reduces potential confusion for both developers and users. This leads to smoother and more intuitive integration into diverse projects.
Choosing a suitable module system impacts how the library is structured and accessed. CommonJS, AMD, and ES modules all offer different advantages and disadvantages. Consider factors like compatibility with different JavaScript environments and build tools when making your choice. The selection of appropriate build tools, like Webpack or Parcel, plays a significant role in optimizing your librarys size and performance. These tools help streamline the build process and ensure your library is efficient for users. Careful consideration is needed for both the development and deployment processes of the library.
The deployment strategy is crucial for accessibility. Publishing your library to npm (Node Package Manager) or similar repositories grants it wider exposure and simplifies installation for others. Consider the licensing implications of your work, choosing a suitable open-source license or proprietary approach that aligns with your goals. Actively engage with the user community, addressing feedback and responding to questions. This fosters a positive reputation and helps improve the library based on real-world usage.
Frequently Asked Questions about Creating a JS Library
Creating and releasing a JavaScript library can involve numerous questions, especially for those new to the process. Understanding the key steps and considerations associated with this process is crucial for building a successful and widely adopted library. Addressing common queries about module systems, testing, and deployment strategies can significantly improve the development experience and ensure the library’s efficiency and usability. Gaining clarity on licensing and version control further solidifies a robust development pipeline.
-
What module system should I use?
The choice depends on your project’s needs and target environment. ES modules are generally preferred for modern projects, offering better compatibility and organization. CommonJS is widely supported but might require transpilation. AMD is an older standard, still used but less common now.
-
How important is testing?
Testing is absolutely crucial. Thorough testing, including unit and integration tests, ensures the librarys functionality, stability, and prevents regressions. Automated testing helps maintain quality and saves time in the long run.
-
How do I deploy my library?
Publish it to a package manager like npm or yarn. This makes it easily installable by others through simple commands. Provide clear instructions on how to install and use your library in your documentation.
-
What about documentation?
Clear, well-written documentation is essential for usability. It should include API references, examples, and explanations of how to use the library’s features. Comprehensive documentation increases adoption rates and makes the library user-friendly.
-
Which license should I choose?
The choice of license depends on your goals. Popular open-source licenses include MIT, GPL, and Apache 2.0. Consider the implications of each license on how others can use and distribute your work.
-
How do I handle versioning?
Use semantic versioning (SemVer) to manage versions and communicate changes to users. This allows for easy understanding of updates and backwards compatibility.
Building a robust JavaScript library demands a methodical approach. Careful planning, starting with defining the library’s precise function and intended use, sets a solid foundation. The subsequent steps modular design, rigorous testing, and clear documentation ensure its quality and usability. This organized approach enables efficient development and maintenance throughout the library’s life cycle. The selection of a suitable module system and version control strategy are also key.
Deployment strategies significantly impact the library’s accessibility and reach. Publishing to a reputable package manager provides ease of access for potential users. Choosing an appropriate open-source license balances sharing your work with protecting your intellectual property. Continuous maintenance and community engagement are critical aspects in maintaining the librarys success and relevance. Regular updates, bug fixes, and incorporating user feedback are essential for long-term viability.
In conclusion, creating a successful and enduring JavaScript library is a rewarding but demanding undertaking. By following best practices, prioritizing robust testing and documentation, and actively managing the librarys evolution, developers can create valuable tools that benefit the broader development community. The benefits of creating your own JS library are numerous, from enhancing productivity and code quality to showcasing your skills and building a professional portfolio.
Therefore, the process of how to create your own JS library is a multifaceted journey, requiring both technical skill and thoughtful planning. The payoff, however, is significant, leading to reusable code, improved efficiency, and a valuable addition to a developer’s skillset and resources.
Youtube Video Reference:
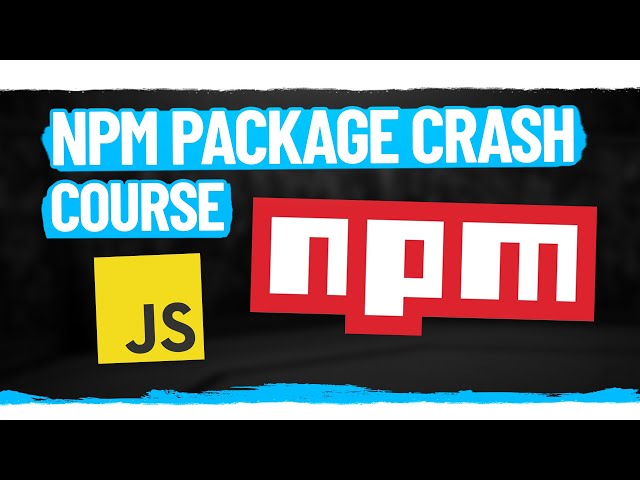