Determining whether an input stream originates from a file is a crucial task in many programming scenarios. The ability to reliably distinguish between file streams and other input sources, such as network connections or in-memory buffers, allows for tailored error handling, optimized resource management, and the implementation of security measures. This capability is essential for robust software design and prevents unexpected behavior caused by incorrect assumptions about the input’s origin. Understanding how to check if input stream is a file provides developers with a powerful tool for creating more resilient and reliable applications. This article will explore various methods and best practices for achieving this.
The need to identify the source of an input stream arises frequently in situations where the application interacts with external data. For instance, a program processing images might need to handle files differently than network streams, adjusting buffer sizes and error-handling strategies accordingly. Similarly, a server receiving data from multiple clients must be capable of identifying the source of each stream to manage resources efficiently and prevent potential security vulnerabilities. Failing to make this distinction can lead to performance degradation, data corruption, or security breaches.
Many programming languages and frameworks provide mechanisms for interacting with input streams in a generic way, abstracting away the underlying source. However, this abstraction can mask important details about the stream’s origin. The methods for determining the source vary depending on the language and the specific libraries used. It is critical to choose an approach that offers the necessary level of reliability and performance for the application’s needs. Furthermore, the process might involve analyzing metadata associated with the stream, employing specific library functions, or resorting to lower-level system calls.
Different operating systems and file systems also introduce nuances into the process of identifying a file stream. The way file metadata is accessed and interpreted can vary significantly. Portability considerations are therefore vital when developing code that needs to work across diverse platforms. A robust solution should account for these variations, providing consistent behavior regardless of the operating system or file system in use. Choosing platform-independent approaches whenever possible is a key aspect of building robust and adaptable software.
How to Check if an Input Stream is a File?
Identifying the source of an input stream is fundamental for building reliable and efficient applications. This process often involves examining properties or metadata associated with the stream. The specific approach varies based on the programming language and environment. However, the core principles remain consistent: determine the stream’s characteristics and compare them to the characteristics expected of a file stream. This typically involves checking for the presence of file-specific attributes or capabilities. Understanding the underlying mechanisms allows developers to create more robust error handling and resource management strategies.
-
Obtain Stream Metadata:
The initial step is retrieving relevant metadata about the input stream. This might include properties such as the stream’s name, type, or associated file descriptor. The method for accessing this information differs significantly depending on the programming language and libraries being used. In some cases, a dedicated function is available; in others, it might necessitate lower-level system calls.
-
Check for File-Specific Attributes:
Once the metadata is obtained, examine it for file-specific indicators. This could involve checking if the stream has attributes associated with files, such as a file path or size. The presence of these attributes strongly suggests that the stream originates from a file. However, absence doesn’t definitively rule it out, as some abstracted streams might lack such attributes.
-
Attempt File Operations:
Attempting file-specific operations, such as seeking to a specific position within the stream, can provide additional confirmation. If the stream supports these operations successfully, it further strengthens the likelihood of it being a file stream. However, a failure doesn’t necessarily negate the possibility; some streams might mimic file behavior to a degree.
-
Examine Underlying File Descriptor (if applicable):
In lower-level programming, examining the underlying file descriptor associated with the stream can definitively determine if it represents a file. The file descriptor is a system-level identifier for an open file. If a valid file descriptor is present and points to a file, it confirms the input stream’s origin. This approach requires a deeper understanding of the operating system’s I/O mechanisms.
Tips for Robustly Determining Input Stream Origin
Successfully identifying if an input stream is a file often requires a multifaceted approach. There is rarely a single, universally applicable technique. Instead, a combination of methods, chosen based on the application’s specific context and the available tools, typically yields the most accurate and reliable results. Robust error handling and graceful degradation are also essential for dealing with situations where the determination is inconclusive.
By combining multiple checks and considering the specific limitations of each technique, developers can increase confidence in the accuracy of their determination. Remember that no single method guarantees absolute certainty; the goal is to maximize confidence based on available information and context. A well-structured approach combines multiple checks, giving precedence to the most reliable methods available.
-
Employ Multiple Checks:
Don’t rely on a single method. Combine several checks for a more reliable result. For instance, check for file-specific attributes, then try file operations, and finally examine the underlying file descriptor if accessible.
-
Handle Exceptions Gracefully:
Anticipate potential errors. Implement robust error handling to gracefully manage situations where attempts to access metadata or perform file operations fail. This prevents unexpected crashes or incorrect behavior.
-
Consider Contextual Information:
Use any available contextual information to aid the determination. If the stream is obtained from a file-opening operation, the likelihood of it being a file stream is considerably higher. This reduces uncertainty when the stream lacks explicit file attributes.
-
Use Appropriate Libraries:
Leverage platform-specific libraries that offer functions for retrieving file metadata or determining stream characteristics. These libraries often provide optimized and reliable methods for this purpose.
-
Prioritize Reliability over Performance (When Necessary):
In security-sensitive applications or situations where accurate identification is paramount, prioritize robust methods even if they might be less efficient. A small performance hit is preferable to security risks or data corruption.
-
Understand OS and Filesystem Differences:
Be aware of the variations in how file systems and operating systems handle file metadata and stream characteristics. This knowledge helps in writing code that is portable and performs reliably across different platforms.
-
Document Assumptions:
Clearly document any assumptions made about the input stream’s origin within the code. This makes the code easier to understand, maintain, and debug. It also assists other developers in understanding the implications of the chosen methods.
The process of distinguishing a file stream from other input sources isn’t always straightforward. The complexity stems from the abstraction layers in modern programming environments, where input streams often present a unified interface regardless of their origin. Therefore, a thorough understanding of the underlying mechanisms and the ability to leverage the available tools are essential for developers to create effective and reliable solutions. Careful consideration of platform-specific behaviors is also vital for building portable and consistent applications.
Furthermore, the need to identify file streams frequently arises in contexts demanding high levels of reliability and security. Incorrect assumptions about the input stream’s source can lead to critical errors or security vulnerabilities. Thus, the strategies implemented must be robust, accounting for potential errors and unexpected situations. Prioritizing both accuracy and error handling is a crucial aspect of developing high-quality software.
In conclusion, robustly determining the source of an input stream is a multifaceted task crucial for reliable software engineering. No single technique guarantees perfect accuracy; instead, developers should employ a combination of methods tailored to the specific context and available tools. Careful consideration of platform variations and comprehensive error handling is essential for ensuring the system’s resilience and security.
Frequently Asked Questions
The challenge of accurately determining whether an input stream originates from a file frequently leads to questions regarding the reliability and limitations of various techniques. Choosing the appropriate approach requires careful consideration of the application’s specific needs and the programming environment’s capabilities.
-
How reliable are the metadata checks?
Metadata checks provide strong clues but aren’t foolproof. Some streams might lack file-specific metadata, even if they originate from a file. Combining metadata checks with other methods increases reliability.
-
What if file operations fail?
Failure of file operations doesn’t necessarily mean the stream isn’t a file. Permissions issues or stream limitations could cause failure. Consider other verification methods if file operations are unsuccessful.
-
Can I always access the underlying file descriptor?
Access to the underlying file descriptor depends on the programming language and environment. Higher-level abstractions might not expose this information directly. Its availability varies significantly.
-
How do I handle streams from different operating systems?
Account for operating system variations in file metadata and stream behavior. Use platform-specific libraries or write portable code that adapts to different operating systems.
-
What are the performance implications of these checks?
The performance impact varies depending on the method used. Simple metadata checks are generally fast, while file operations or low-level system calls might be slower. Balance reliability with performance needs.
-
Are there security considerations?
In security-sensitive applications, prioritize reliability over speed. Insecure assumptions about input streams could lead to vulnerabilities. Thoroughly validate all input sources.
Understanding the nuances of input stream identification is vital for creating robust and secure applications. The methods discussed offer a framework for addressing this challenge, but the optimal approach depends significantly on the specific context.
Remember that the reliability of these methods relies on careful implementation and consideration of potential errors. Thorough testing and validation are crucial to ensuring the accuracy and robustness of the implementation. The choice of method should also align with the applications sensitivity to performance and security requirements.
Ultimately, the goal is to build applications that handle input streams reliably and safely, regardless of their origin. Choosing the appropriate techniques and implementing them carefully are fundamental aspects of building high-quality software.
In conclusion, the ability to reliably determine how to check if input stream is a file is a cornerstone of robust software design. By employing a multifaceted approach and carefully considering the potential pitfalls, developers can create applications that handle diverse input sources effectively and securely.
Youtube Video Reference:
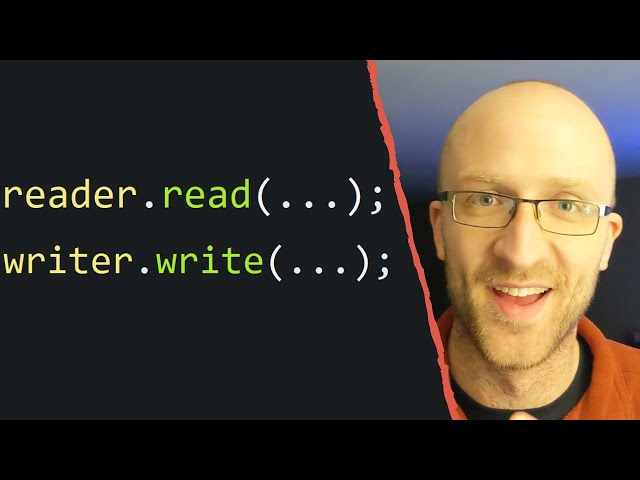