Determining the existence of a directory before attempting file operations is crucial for robust C programming. In C programming, how to check if a folder exists is a fundamental task, preventing errors and improving the overall reliability of applications. This capability is essential for numerous tasks, ranging from simple file management to complex data processing. Incorrectly handling directory existence can lead to program crashes or unexpected behavior. Therefore, understanding the methods for verifying directory presence is a key skill for any C programmer. This article explores various techniques and best practices for achieving this critical functionality.
The ability to check for a directory’s existence in C is paramount for creating secure and efficient applications. Failing to perform this check can lead to runtime errors, potentially compromising data integrity or causing unexpected program termination. This functionality is particularly important when dealing with user-provided paths or dynamically generated directory structures. By proactively checking for the directory’s existence, developers can prevent these issues and write more robust code. The techniques discussed in this article provide developers with a powerful tool for building reliable and stable software.
Many applications rely on the correct handling of file paths and directories. A program that attempts to write to a non-existent folder will invariably fail, causing frustration for the user and potentially data loss. The functionality to detect the absence of a folder allows the program to handle this situation gracefully, perhaps by creating the directory or providing a user-friendly error message. Effective error handling is central to good software design, and directory existence checks form a critical part of this process. The implementation of this check demonstrates a commitment to building robust and reliable software.
Efficiently checking for directory existence also contributes to the overall performance of a program. Unnecessary attempts to access non-existent directories consume valuable system resources and slow down execution. By first verifying the presence of a directory, a program can avoid wasted cycles, leading to a more responsive and efficient user experience. Furthermore, this check can minimize potential security risks associated with unauthorized access attempts. Therefore, directory existence checks are not only crucial for correctness but also for performance optimization.
In C programming, how to check if a folder exists?
Verifying the existence of a directory within a C program involves utilizing system calls to interact with the underlying operating system. The most common approach involves employing the `stat()` function, a powerful tool that provides comprehensive information about a file or directory. Alternatively, the `opendir()` function can be used, which specifically handles directory access. Both functions, when used correctly, provide reliable methods for determining whether a specified directory path exists. The choice between these functions often depends on the broader context of the program and whether additional file information is required beyond just the existence check. Understanding the nuances of each approach is crucial for effective implementation.
-
Using `stat()`
The `stat()` function retrieves information about a file or directory. If the path specified does not exist, `stat()` will return a non-zero value, indicating failure. Conversely, a successful call with a zero return value confirms the path’s existence. Remember to include the “ header file for access to the `stat()` function and its associated data structures. Error handling is crucial to ensure robust operation.
-
Using `opendir()`
The `opendir()` function attempts to open a directory. A successful call returns a directory stream pointer; failure results in a `NULL` pointer. This function provides a more direct way to check for directory existence, and it’s particularly useful when subsequent operations will require interaction with the directory contents. Remember to include “ for this function and to close the directory stream using `closedir()` after use to release resources.
-
Error Handling
Regardless of the chosen method, comprehensive error handling is crucial. Check for errors returned by `stat()` and `opendir()` and handle them appropriately. This might involve logging errors, providing informative messages to the user, or taking alternative actions. Neglecting error handling can lead to unpredictable program behavior and potential instability.
Tips for Effectively Checking for Directory Existence
While the `stat()` and `opendir()` functions provide the core functionality, several best practices enhance the reliability and efficiency of directory existence checks. These include careful error handling, the use of absolute paths whenever possible, and consideration of platform-specific variations in path separators. Implementing these tips will lead to more robust and portable code.
Using these techniques effectively is critical for ensuring the integrity and security of the application. Proper error handling is essential to prevent unexpected behavior or security vulnerabilities that might arise from improperly checking directory existence. Thorough testing is necessary to cover all potential scenarios, including cases where paths might be invalid or incomplete.
-
Use Absolute Paths:
Absolute paths eliminate ambiguity and avoid potential issues with relative paths. An absolute path always begins from the root directory, ensuring consistent interpretation across different contexts.
-
Robust Error Handling:
Always check the return value of `stat()` and `opendir()` for errors. Handle errors gracefully, either by providing informative error messages or taking corrective actions, such as creating the missing directory.
-
Platform-Specific Considerations:
Account for differences in path separators (e.g., ‘/’ on Unix-like systems, ‘\’ on Windows) to ensure portability. Consider using platform-specific macros or functions to handle path normalization.
-
Normalize Paths:
Before performing the existence check, normalize the path to remove redundant separators or unnecessary components. This can improve consistency and prevent unexpected failures due to improperly formatted paths.
-
Avoid Race Conditions:
If the directory creation might happen concurrently, employ mechanisms like atomic operations or file locking to prevent race conditions. These safeguards ensure that the existence check accurately reflects the current state of the file system.
The choice between using `stat()` and `opendir()` depends on the specific needs of the application. If only the existence of the directory needs to be determined, `stat()` is often sufficient. However, if further interaction with the directory’s contents is required, `opendir()` provides a more direct approach, streamlining subsequent operations. Consistent error handling should always be a priority, regardless of the chosen method.
Efficiently checking for directory existence not only prevents errors and improves program robustness but also contributes to overall performance. Avoiding unnecessary attempts to access non-existent directories saves processing time and resources. Moreover, this practice contributes to a more responsive and efficient user experience by minimizing delays and potential program crashes.
Remember that security is paramount. Improper handling of file paths and directories can lead to vulnerabilities. By carefully checking for directory existence and implementing robust error handling, developers can significantly enhance the security of their applications. This proactive approach is an essential element of secure software development practices.
Frequently Asked Questions about Checking for Directory Existence
Several common questions arise regarding directory existence checks in C. Understanding the answers to these questions can help developers avoid common pitfalls and implement effective solutions.
-
What happens if the path is invalid?
If the provided path is invalid (e.g., contains illegal characters or points to a non-existent location), both `stat()` and `opendir()` will return an error. Proper error handling is crucial to manage these situations and prevent program crashes.
-
How can I handle permission errors?
Permission errors occur if the program lacks sufficient privileges to access the specified directory. These errors will also be indicated by the return values of `stat()` and `opendir()`. The program should handle these errors gracefully, perhaps by providing an informative error message to the user.
-
Is there a platform-independent way to check for directory existence?
While there’s no single platform-independent function, careful use of `stat()` along with appropriate handling of path separators and error conditions can produce highly portable code. Using a cross-platform library could also simplify this task.
-
What are the performance implications of repeatedly checking for directory existence?
Repeatedly checking can impact performance. Cache the result if possible, or restructure the program to minimize redundant checks. In scenarios where the directory’s existence is unlikely to change, caching is a highly effective optimization strategy.
-
How can I make my directory existence check code more robust?
Thorough testing, comprehensive error handling, and the use of absolute paths are key components of robust code. Consider using static analysis tools to identify potential issues and further enhance code reliability.
In summary, robustly determining whether a directory exists in a C program is a vital aspect of secure and efficient software development. The techniques outlined in this article provide the necessary foundation for building reliable applications that gracefully handle potential errors and improve the overall user experience. Consistent application of these methods demonstrates a commitment to quality software engineering practices.
The use of either `stat()` or `opendir()`, combined with comprehensive error handling and best practices, forms a powerful toolkit for managing directories effectively in C programs. Understanding the nuances of these functions is a significant step towards creating more sophisticated and robust software.
Mastering directory existence checks is a cornerstone of proficiency in C programming. The ability to reliably and efficiently ascertain a directorys presence is not merely a technical detail, but a fundamental skill that elevates the quality and stability of any C-based software project. Careful consideration of error handling and platform-specific issues is essential for producing portable and reliable code.
Therefore, understanding how to effectively determine directory existence is a crucial skill for any C programmer, contributing significantly to the robustness and reliability of their applications. Proper application of the techniques and best practices outlined in this article will result in more efficient, secure, and user-friendly software.
Youtube Video Reference:
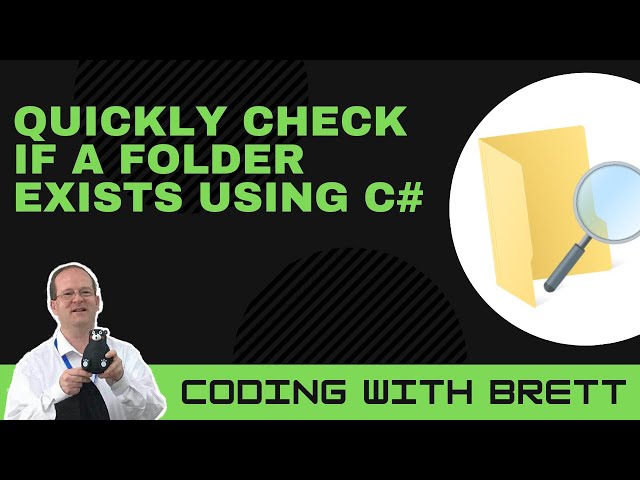